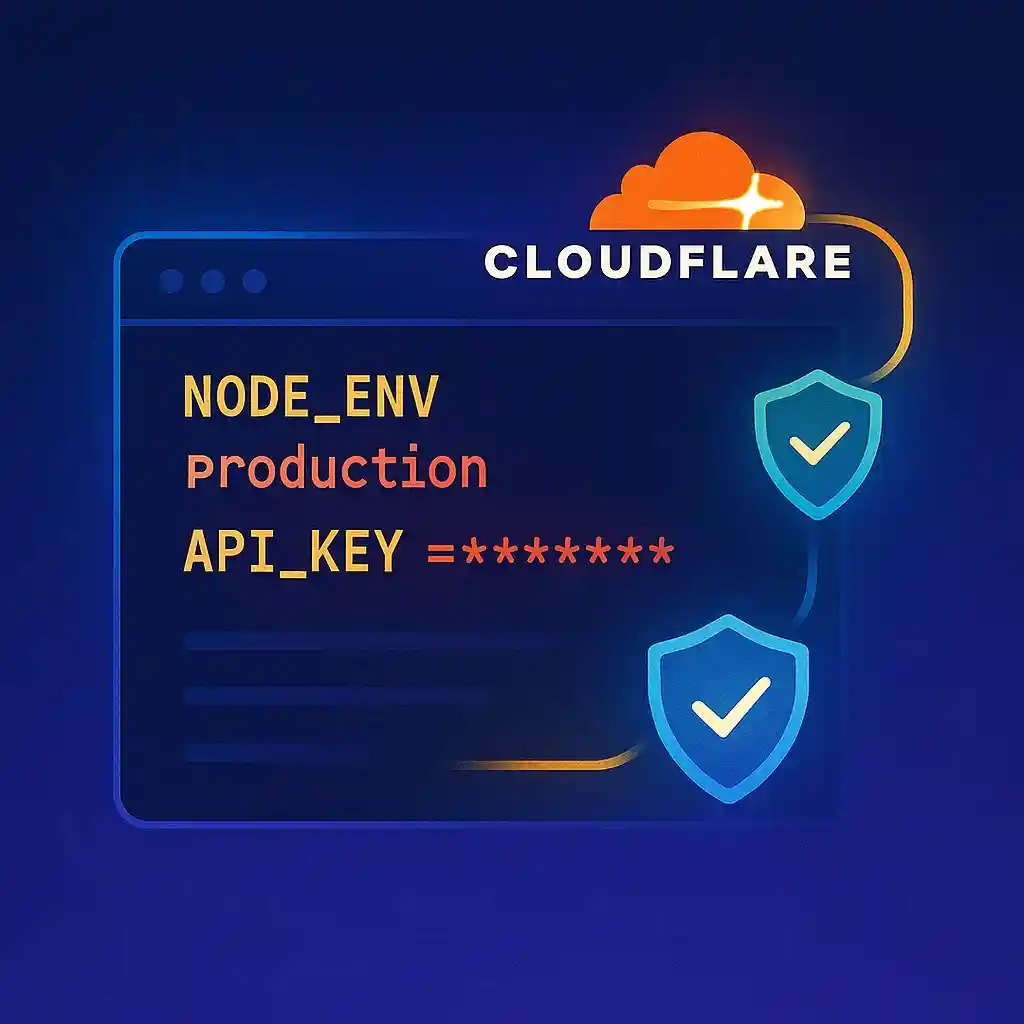
Understanding Environment Variables in AstroJS
With Astro 5 environment variables got a huge upgrade in developer experience and safety. Until recently, AstroJS developers using Cloudflare had to use the legacy environment variable handling system. The good news is that Astro’s new environment variable configuration system now works seamlessly with the Cloudflare adapter!
You can always check out the AstroJS docs for the latest information, but this guide focuses specifically on the Cloudflare integration details you need to know.
Legacy Environment Variable Handling: The Old Way
Let’s quickly review the previous method of handling environment variables with Cloudflare. This context will help you understand why the new approach is such a significant improvement.
With the legacy approach, you had to manually define your environment variables and inject them into the build process:
import { defineConfig } from 'astro/config';import cloudflare from '@astrojs/cloudflare';
const envVars = ['PUBLIC_VARIABLE_1', 'PUBLIC_VARIABLE_2', 'PRIVATE_VARIABLE_3'] as const;
export default defineConfig({ site: 'https://example.com', output: 'static', trailingSlash: 'ignore', integrations: [], adapter: cloudflare(), vite: { ssr: { target: 'webworker', }, define: envVars.reduce((acc, key) => { acc[`process.env.${key}`] = JSON.stringify(process.env[key]); return acc; }, {}), },});
You also needed to manually define the types for your environment variables:
type Variables = 'PUBLIC_VARIABLE_1' | 'PUBLIC_VARIABLE_2' | 'PRIVATE_VARIABLE_3';
interface ImportMetaEnv extends Record<Variables, string> {}
interface ImportMeta { readonly env: ImportMetaEnv;}
Then you could access your variables like this:
import.meta.env.PUBLIC_VARIABLE_1;
This approach had several significant drawbacks:
- No security boundary: Private variables could accidentally be exposed to the client
- No validation: Missing environment variables wouldn’t cause errors until runtime
- Limited type safety: TypeScript couldn’t verify the correct usage of variables
- No default values: You couldn’t specify fallbacks for optional variables
- Manual maintenance: You had to update multiple files when adding new variables
Limited Time Launch Sale
Skip the configuration headaches! Our AstroJS starter template will save you hours of setup time and potential security issues.
GET 60% OFF!Modern Environment Variable System: Type-Safe and Secure
Astro’s new environment variable system addresses all these limitations with an elegant, type-safe solution that now works perfectly with Cloudflare. Let’s explore the benefits and implementation details.
Key Benefits of the New Environment Variable System
-
Runtime validation: Errors if required variables are missing
-
Enhanced type safety: Full TypeScript integration with proper types
-
Intelligent IntelliSense: Autocomplete in your IDE for available variables
-
Security boundaries: Private variables cannot be exposed to the client
-
Default values: Specify fallbacks for optional variables
-
Centralized configuration: Define everything in one place
How to Implement the New Environment Variable System
import { defineConfig, envField } from 'astro/config';import cloudflare from '@astrojs/cloudflare';
// https://astro.build/configexport default defineConfig({ site: 'https://example.com', output: 'static', trailingSlash: 'ignore', integrations: [], adapter: cloudflare(), env: { schema: { // Public variable accessible anywhere PUBLIC_VARIABLE_1: envField.string({ context: 'client', access: 'public', default: 'default value', }), // Public variable accessible on the server PUBLIC_VARIABLE_2: envField.boolean({ context: 'server', access: 'public', default: false, }), // Private variable accessible on the server PRIVATE_VARIABLE_3: envField.number({ context: 'server', access: 'secret', }), }, }, vite: { ssr: { target: 'webworker', }, },});
Notice how each variable is defined with:
- Type information (string, boolean, number)
- Context (client or server)
- Access level (public or secret)
- Optional default values
This configuration automatically generates the appropriate TypeScript types and enforces security boundaries between client and server code.
How to Access Environment Variables in Your Code
// Server-side codeimport { PUBLIC_VARIABLE_2, PRIVATE_VARIABLE_3 } from 'astro:env/server';
// Client-side codeimport { PUBLIC_VARIABLE_1 } from 'astro:env/client';
This import pattern creates a clear separation between client and server variables, preventing accidental exposure of sensitive information. The TypeScript compiler will even throw errors if you try to import server variables in client code!
Setting Up Cloudflare for AstroJS Environment Variables
For the new environment variable system to work correctly with Cloudflare, you need to update your Cloudflare Pages settings. You have two options:
- Set the compatibility date to April 1, 2025, or later
- Enable the compatibility flag specifically for this feature
Here’s how to access these settings in your Cloudflare dashboard:
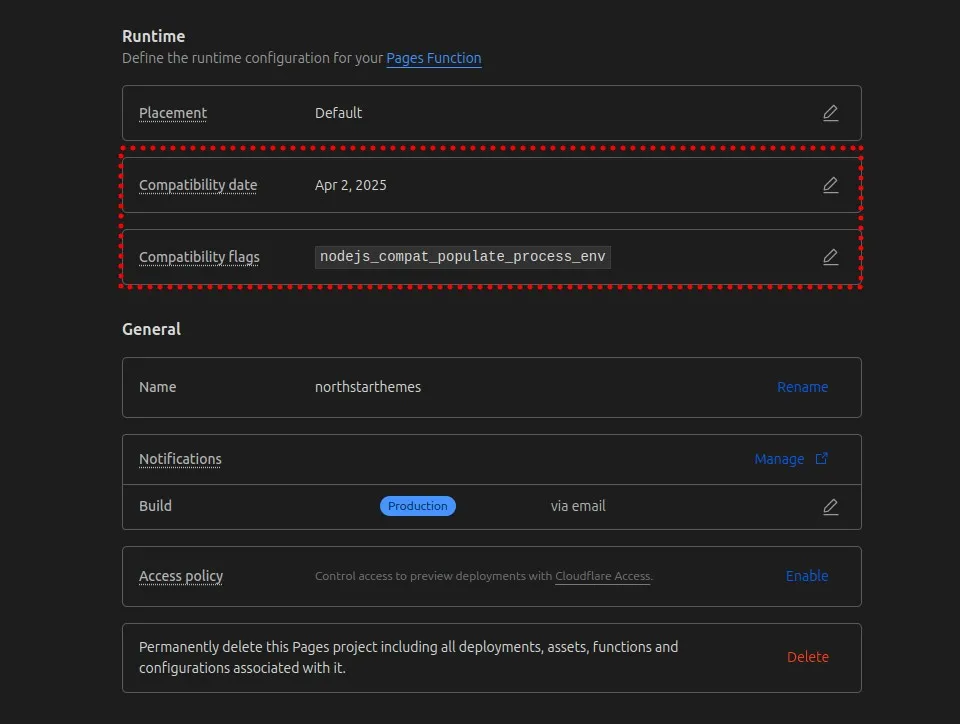
Option 1: Updating Your Compatibility Date
If you’re starting a new project or can safely update your compatibility date:
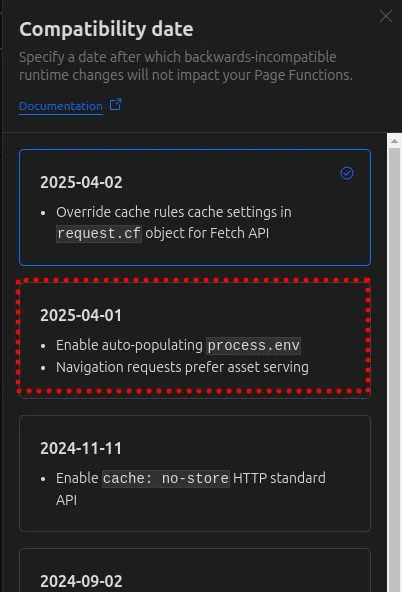
Option 2: Enabling the Specific Compatibility Flag
If you need to maintain an earlier compatibility date for other features:
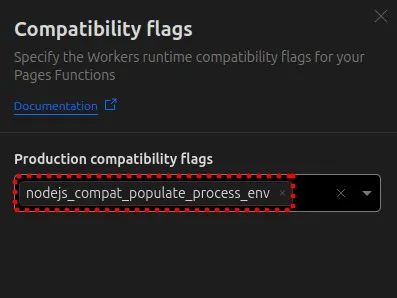
Environment Variable Best Practices for AstroJS and Cloudflare
-
Use descriptive variable names that indicate their purpose
-
Set appropriate access levels (public vs. secret) for each variable
-
Provide default values for non-critical configuration
-
Group related variables together in your schema
-
Document expected formats in comments for team members
By following these practices, you’ll create a more maintainable, secure, and developer-friendly configuration for your AstroJS projects on Cloudflare Pages.
The new environment variable system represents a significant improvement in how we manage configuration in AstroJS projects. With proper Cloudflare integration, you can now enjoy all these benefits while deploying to one of the fastest and most reliable edge networks available.
Related Articles
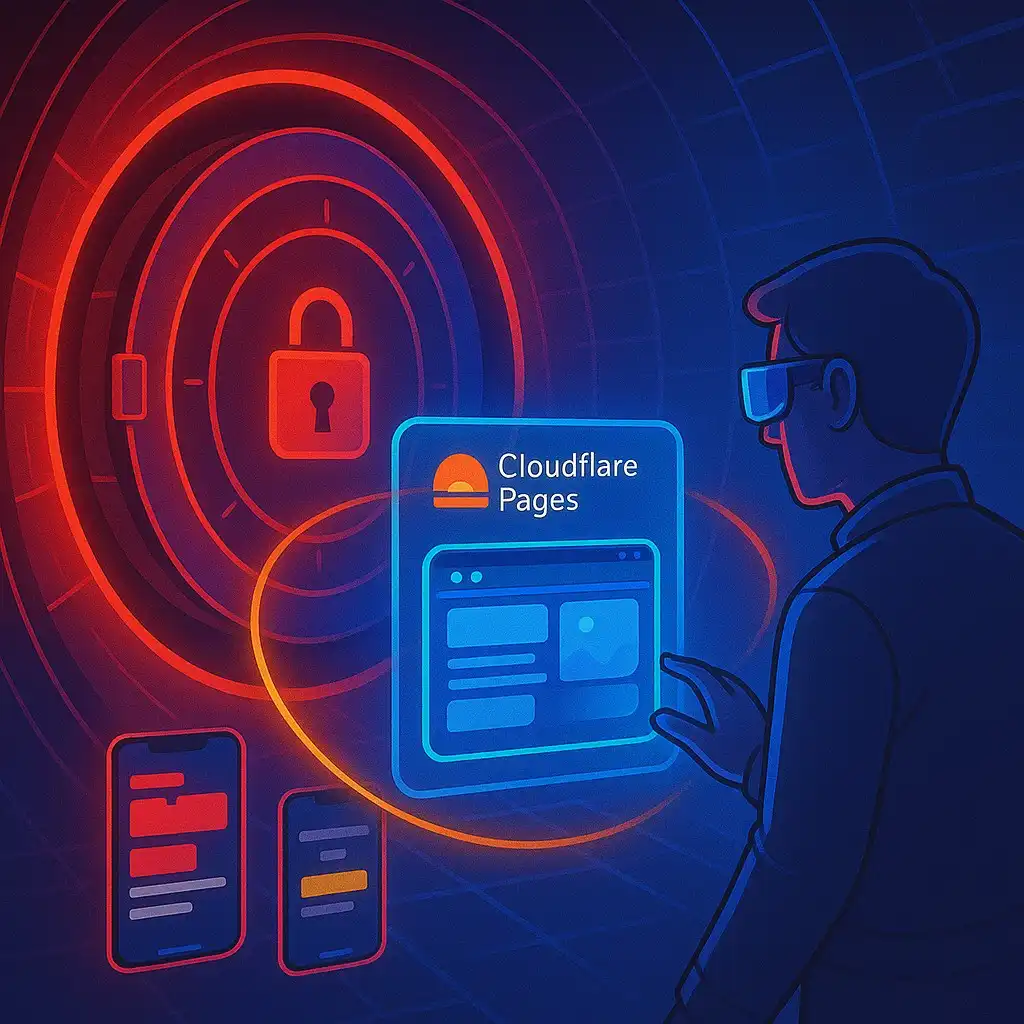
How To Create A Secure Staging Environment For Cloudflare Pages
Testing website changes before they go live is essential for maintaining a professional online presence. Learn how to create a secure, password-protected staging environment for your Cloudflare Pages site.
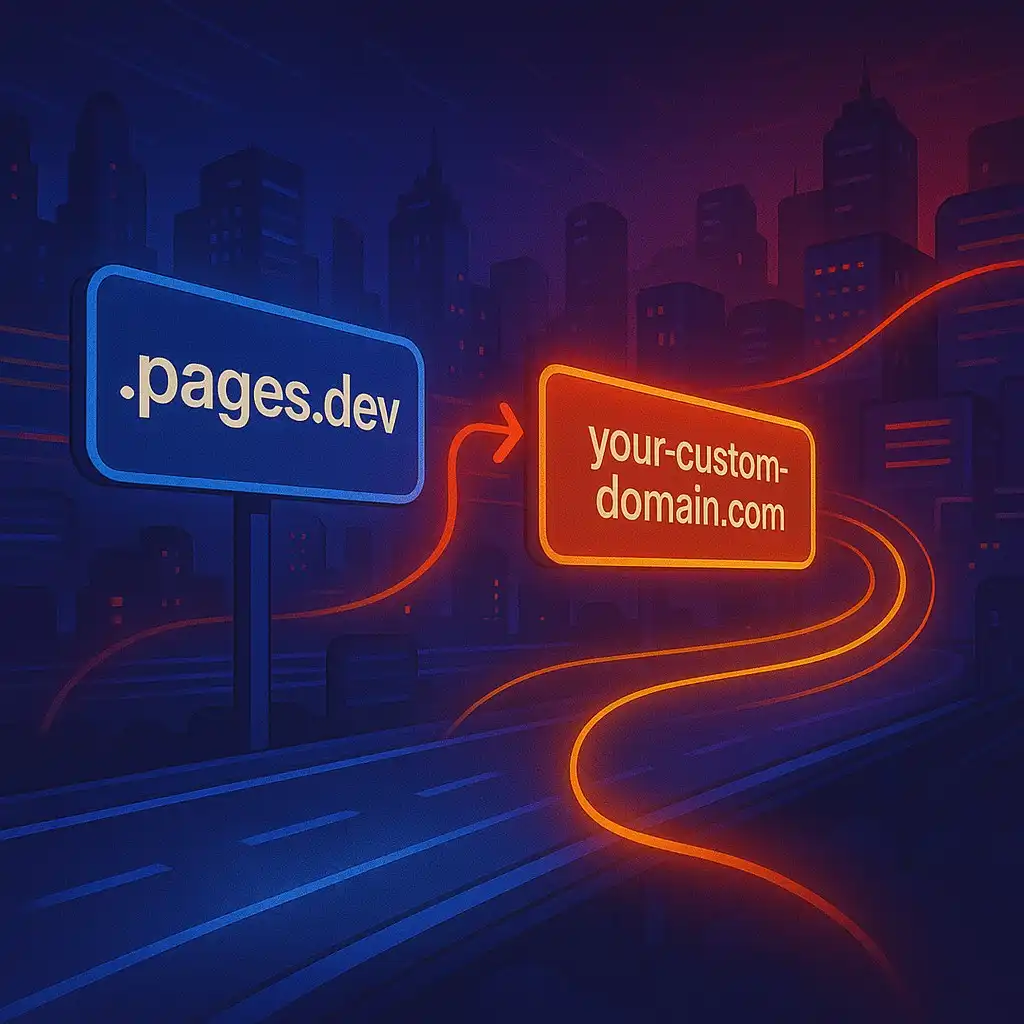
How To Redirect .pages.dev to Your Domain
Learn how to redirect .pages.dev to your domain with this step-by-step guide
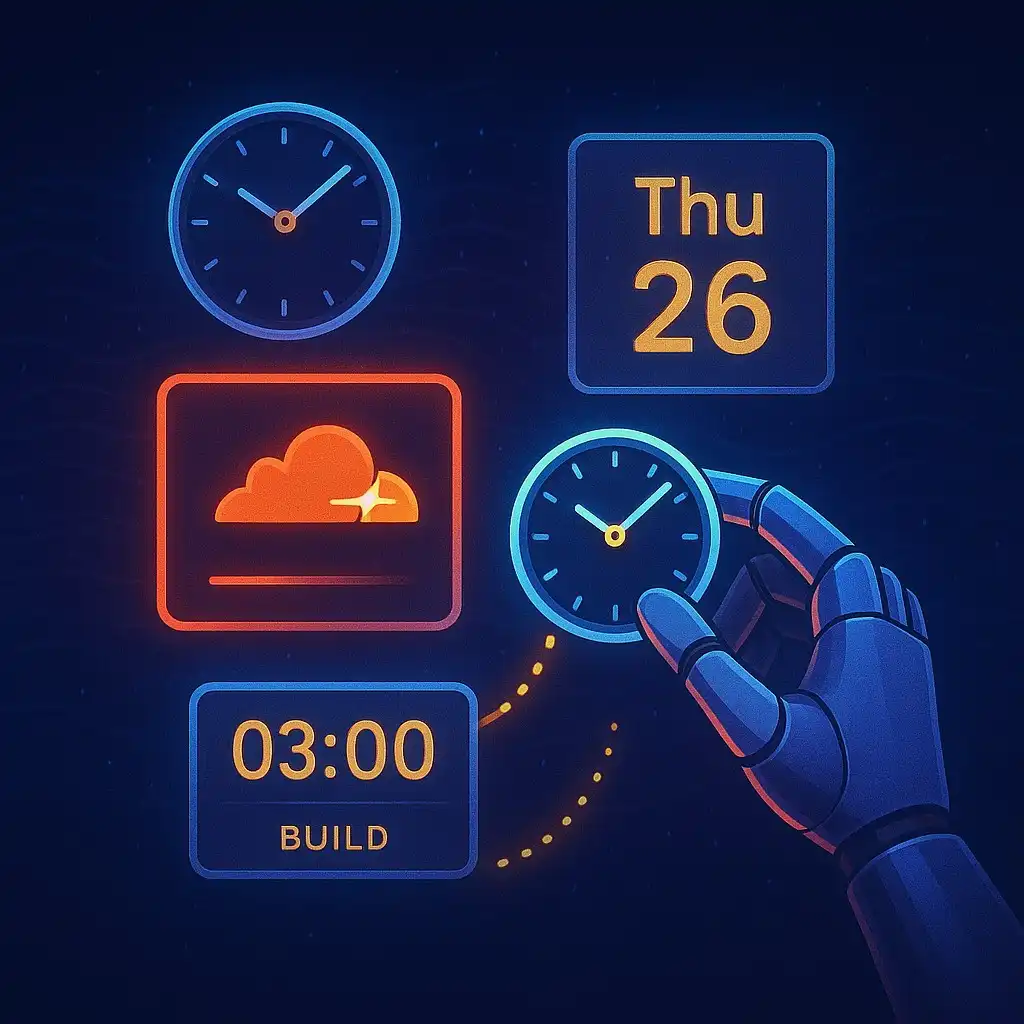
Schedule Cloudflare Pages Builds
Schedule your Cloudflare Pages builds to run at specific times. No external services needed.
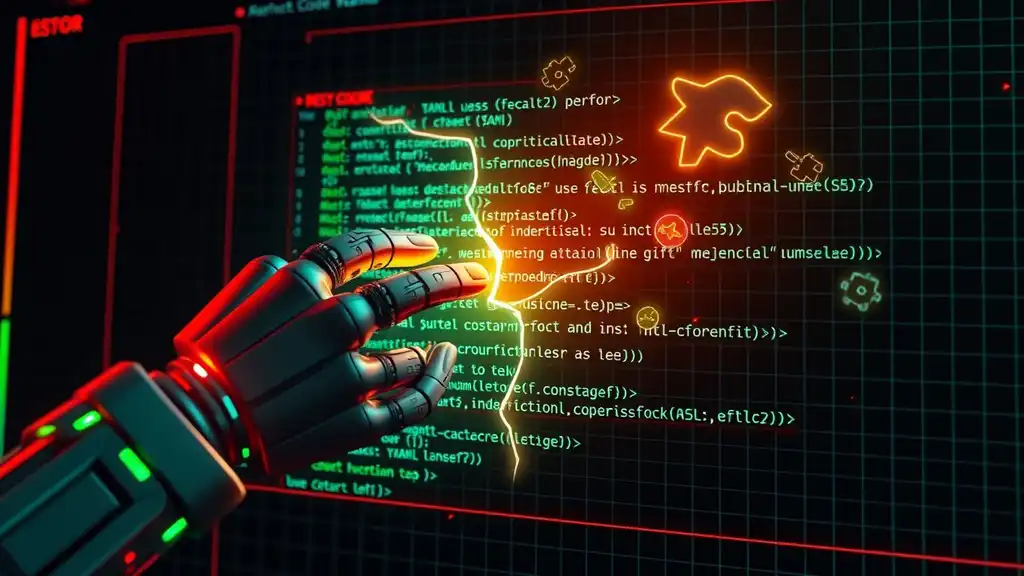
Fix Bad Indentation Of A Mapping Entry Error In Astro
Troubleshoot and resolve the common "bad indentation of a mapping entry" error in AstroJS with this practical guide.